Autodesk.Revit.DB
Autodesk.Revit.DB is a namespace that is available to you in the python script node. With it you can access alot of functions for handling geometry and Reit elements like Arc, Circle, Area, Room, etc).
The link to this namespace is http://www.revitapidocs.com/2017/87546ba7-461b-c646-cbb1-2cb8f5bff8b2.htm.
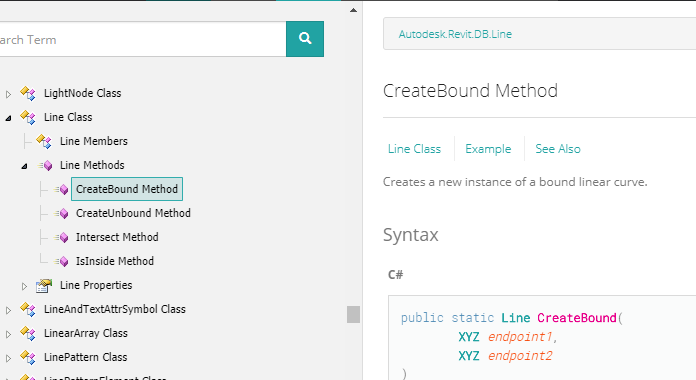
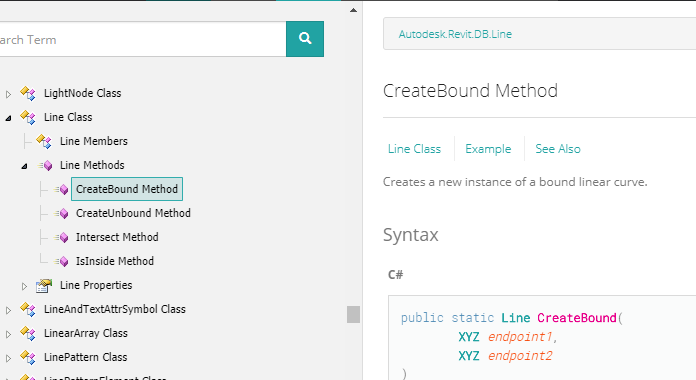
Let’s say you want to create a Revit line. This is part of the Autodesk.Revit.DB namespace and if you navigate to it’s methods you will see the Create method. Notice here that it accepts XYZ values and remember that Revit internally works in feet so it’s up to you to do the conversions.
import clr clr.AddReference("RevitAPI"); from Autodesk.Revit.DB import XYZ as XYZ from Autodesk.Revit.DB import Line as Line #create XYZ points (in feet) startPointXYZ = XYZ(0,0,0) endPointXYZ = XYZ(10,10,10) #create line line = Line.CreateBound(startPointXYZ, endPointXYZ) # Assign your output to the OUT variable. OUT = line
Transaction
A transaction is a context required in order to make any changes to a Revit model. The easy way to do this in Dynamo Python is to use the TransactionManager. For this you have to import into you graph the dll RevitServices.
This file is installed with the installation of Dynamo and it’s located under <drive>:\Program Files\Dynamo\Dynamo Revit\1.3\Revit_<version>


There are some utilities available here like in the namespace Elements you have some query functions, but the most important part is the transaction manager and this one allows you to make changes to model elements.
You can reference RevitServices by using the clr method AddReference
import clr import RevitServices from RevitServices.Persistence import DocumentManager from RevitServices.Transactions import TransactionManager doc = DocumentManager.Instance.CurrentDBDocument TransactionManager.Instance.EnsureInTransaction(doc) #your statements here TransactionManager.Instance.TransactionTaskDone(doc)
Document, GetElement
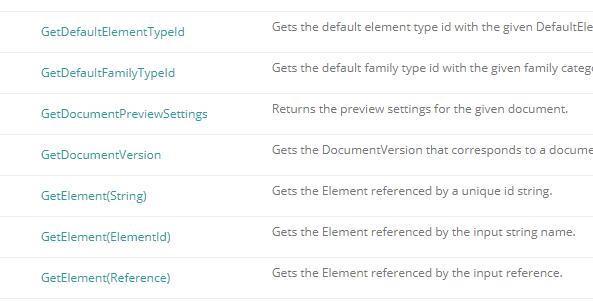
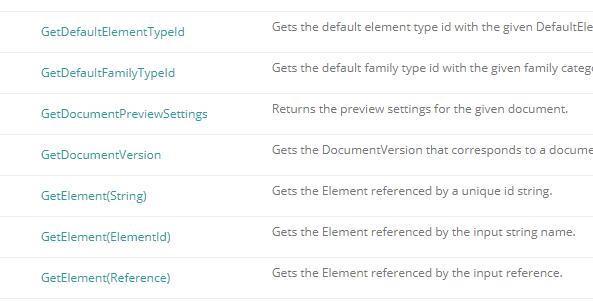
Also from the RevitServices you can have access to the active document. This is also part of the Autodesk.Revit.DB namespace and RevitServices provides it as a link to your Revit Model Active Document.
You can use several properties that are really helpfull. Some usefull properties are FamilyCreate, FamilyManager, Phases, Title. Etc.
It also has alot of usefull methods and one of the method that I use the most is GetElement(). It’s used to retrieve a Revit element by the id.
elementIdInteger = IN[0] import clr clr.AddReference("RevitAPI"); from Autodesk.Revit.DB import ElementId as ElementId clr.AddReference("RevitServices") import RevitServices from RevitServices.Persistence import DocumentManager doc = DocumentManager.Instance.CurrentDBDocument #create a new instance of ElementId elementId = ElementId(elementIdInteger) # Assign your output to the OUT variable. OUT = doc.GetElement(elementId)
Settings


The settings object provides access to general components of the Autodesk Revit application, such as Categories.
http://www.revitapidocs.com/2017/9aa29bb7-d720-8c97-0ccb-e3e6046c545c.htm
import clr clr.AddReference("RevitServices") import RevitServices from RevitServices.Persistence import DocumentManager doc = DocumentManager.Instance.CurrentDBDocument categories = doc.Settings.Categories OUT = categories